Chapter 4 Basic Reactivity
Exercise 4.3.6.1
Draw the reactive graph for the following server functions:
<- function(input, output, session) {
server1 <- reactive(input$a + input$b)
c <- reactive(c() + input$d)
e $f <- renderText(e())
output
}
<- function(input, output, session) {
server2 <- reactive(input$x1 + input$x2 + input$x3)
x <- reactive(input$y1 + input$y2)
y $z <- renderText(x() / y())
output
}
<- function(input, output, session) {
server3 <- reactive(c() ^ input$d)
d <- reactive(input$a * 10)
a <- reactive(b() / input$c)
c <- reactive(a() + input$b)
b }
Solution.
Solution
To create the reactive graph we need to consider the inputs, reactive expressions, and outputs of the app.
For server1
we have the following objects:
- inputs:
input$a
,input$b
, andinput$d
- reactives:
c()
ande()
- outputs:
output$f
Inputs input$a
and input$b
are used to create c()
, which is combined with
input$d
to create e()
. The output depends only on e()
.
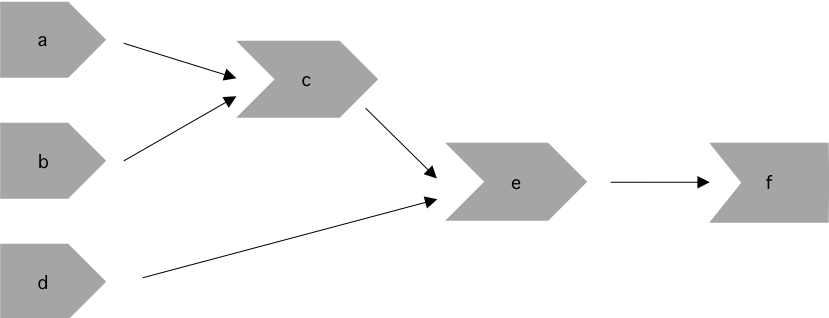
reactive graph - server 1
For server2
we have the following objects:
- inputs:
input$y1
,input$y2
,input$x1
,input$x2
,input$x3
- reactives:
y()
andx()
- outputs:
output$z
Inputs input$y1
and input$y2
are needed to create the reactive y()
. In
addition, inputs input$x1
, input$x2
, and input$x3
are required to create
the reactive x()
. The output depends on both x()
and y()
.
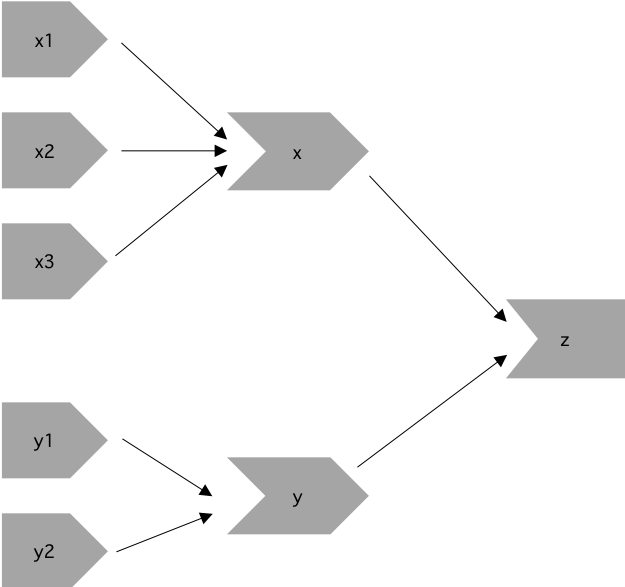
reactive graph - server 2
For server3
we have the following objects:
- inputs:
input$a
,input$b
,input$c
,input$d
- reactives:
a()
,b()
,c()
,d()
As we can see below, a()
relies on input$a
, b()
relies on both a()
and
input$b
, and c()
relies on both b()
and input$c
. The final output
depends on both c()
and input$d
.
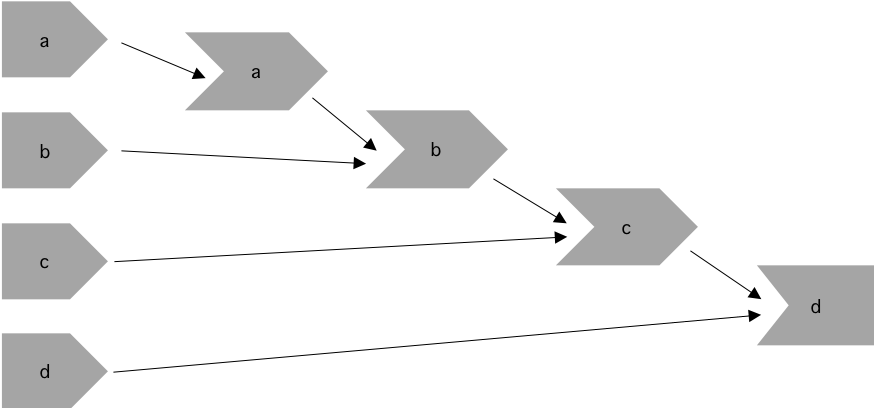
reactive graph - server 3
Exercise 4.3.6.2
Why will this code fail?
<- reactive(df[input$var])
var <- reactive(range(var(), na.rm = TRUE)) range
Why is var()
a bad name for a reactive?
Solution.
Solution
This code doesn’t work because we called our reactive range
, so when we call the range
function we’re actually calling our new reactive. If we change the name of the reactive from range
to col_range
then the code will work. Similarly, var()
is not a good name for a reactive because it’s already a function to compute the variance of x
! ?cor::var
library(shiny)
<- mtcars
df
<- fluidPage(
ui selectInput("var", NULL, choices = colnames(df)),
verbatimTextOutput("debug")
)
<- function(input, output, session) {
server <- reactive( df[input$var] )
col_var <- reactive({ range(col_var(), na.rm = TRUE ) })
col_range $debug <- renderPrint({ col_range() })
output
}
shinyApp(ui = ui, server = server)